React Bootstrap Tags, Labels & Badges
React Bootstrap Tags, Labels & Badges - Bootstrap 4 & Material Design
Note: We are transitioning MDB4 to a legacy version and focusing on developing MDB5.
While we'll continue to support for the transition period, we encourage you to migrate to
MDB5. We're offering a 50% discount on MDB5 PRO to help with your transition,
enabling you to leverage the full potential of the latest version. You can find more information here.
get 50% discount on MDB5 PRO
Their main goal is to provide your visitors with an intuitive way of getting what they want. Just consider, how convenient it is to find all the articles related to web development just by using web development tag.
Basic example
Badges scale to match the size of the immediate parent element by using relative font sizing and em
units.
import React from "react";
import { MDBBadge, MDBContainer } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<h1>This is the first heading <MDBBadge color="primary">New</MDBBadge>
</h1>
<h2>This is the second heading <MDBBadge color="primary">New</MDBBadge>
</h2>
<h3>This is the third heading <MDBBadge color="primary">New</MDBBadge>
</h3>
<h4>This is the fourth heading <MDBBadge color="primary">New</MDBBadge>
</h4>
<h5>This is the fifth heading <MDBBadge color="primary">New</MDBBadge>
</h5>
<h6>This is the sixth heading <MDBBadge color="primary">New</MDBBadge>
</h6>
</MDBContainer>
);
};
export default BadgePage;
Badges can be used as part of links or buttons to provide a counter.
import React from "react";
import { MDBBadge, MDBContainer, MDBBtn } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBtn color="primary">
Notifications <MDBBadge color="danger" className="ml-2">4</MDBBadge>
</MDBBtn>
</MDBContainer>
);
};
export default BadgePage;
Note that depending on how they are used, badges may be confusing for users of screen readers and similar assistive technologies. While the styling of badges provides a visual cue as to their purpose, these users will simply be presented with the content of the badge. Depending on the specific situation, these badges may seem like random additional words or numbers at the end of a sentence, link, or button.
Unless the context is clear (as with the “Notifications” example, where it is understood that the “4” is the number of notifications), consider including additional context with a visually hidden piece of additional text.
import React from "react";
import { MDBBadge, MDBContainer, MDBBtn } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBtn color="primary">
Profile <MDBBadge color="danger" className="ml-2">9</MDBBadge>
<span className="sr-only">unread messages</span>
</MDBBtn>
</MDBContainer>
);
};
export default BadgePage;
Contextual variations
Add any of the below mentioned modifier classes to change the appearance of a badge.
import React from "react";
import { MDBBadge, MDBContainer } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBadge color="default">Default</MDBBadge>
<MDBBadge color="primary">Primary</MDBBadge>
<MDBBadge color="success">Success</MDBBadge>
<MDBBadge color="info">Info</MDBBadge>
<MDBBadge color="warning">Warning</MDBBadge>
<MDBBadge color="danger">Danger</MDBBadge>
<MDBBadge color="light">Light</MDBBadge>
<MDBBadge color="dark">Dark</MDBBadge>
</MDBContainer>
);
};
export default BadgePage;
Conveying meaning to assistive technologies
Using color to add meaning only provides a visual indication, which will not be conveyed to users of assistive technologies – such as screen readers. Ensure that information denoted by the color is either obvious from the content itself (e.g. the visible text), or is included through alternative means, such as additional text hidden with the
.sr-only
class.
Pill badges
Use the pill
prop to make badges more rounded (with a larger border-radius
and additional horizontal padding
).
import React from "react";
import { MDBBadge, MDBContainer } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBadge pill color="default">Default</MDBBadge>
<MDBBadge pill color="primary">Primary</MDBBadge>
<MDBBadge pill color="success">Success</MDBBadge>
<MDBBadge pill color="info">Info</MDBBadge>
<MDBBadge pill color="warning">Warning</MDBBadge>
<MDBBadge pill color="danger">Danger</MDBBadge>
<MDBBadge pill color="light">Light</MDBBadge>
<MDBBadge pill color="dark">Dark</MDBBadge>
</MDBContainer>
);
};
export default BadgePage;
Links
Using the contextual badges with an <a>
tag quickly provide actionable
badges with hover and focus states.
import React from "react";
import { MDBBadge, MDBContainer } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBadge tag="a" color="default">Default</MDBBadge>
<MDBBadge tag="a" color="primary">Primary</MDBBadge>
<MDBBadge tag="a" color="success">Success</MDBBadge>
<MDBBadge tag="a" color="info">Info</MDBBadge>
<MDBBadge tag="a" color="warning">Warning</MDBBadge>
<MDBBadge tag="a" color="danger">Danger</MDBBadge>
<MDBBadge tag="a" color="light">Light</MDBBadge>
<MDBBadge tag="a" color="dark">Dark</MDBBadge>
</MDBContainer>
);
};
export default BadgePage;
Badges with icons
import React from "react";
import { MDBBadge, MDBContainer, MDBIcon } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBBadge color="default">
<MDBIcon fab icon="facebook-f" />
</MDBBadge>
<MDBBadge color="primary">
<MDBIcon fab icon="instagram" />
</MDBBadge>
<MDBBadge color="success">
<MDBIcon fab icon="snapchat-ghost" />
</MDBBadge>
<MDBBadge color="info">
<MDBIcon icon="anchor" />
</MDBBadge>
<MDBBadge color="warning">
<MDBIcon far icon="sun" />
</MDBBadge>
<MDBBadge color="danger">
<MDBIcon icon="battery-three-quarters" />
</MDBBadge>
<MDBBadge color="pink">
<MDBIcon icon="wheelchair" />
</MDBBadge>
<MDBBadge color="light-blue">
<MDBIcon far icon="heart" />
</MDBBadge>
<MDBBadge color="purple">
<MDBIcon icon="bullhorn" />
</MDBBadge>
<MDBBadge color="orange">
<MDBIcon fab icon="btc" />
</MDBBadge>
<MDBBadge color="badge-pill">
<MDBIcon far icon="comments" />
</MDBBadge>
<MDBBadge color="orange">
<MDBIcon icon="coffee" />
</MDBBadge>
<MDBBadge color="green">
<MDBIcon icon="user" />
</MDBBadge>
<MDBBadge color="indigo">
<MDBIcon fab icon="android" size="2x" />
</MDBBadge>
<MDBBadge color="cyan">
<MDBIcon icon="cog" size="2x" />
</MDBBadge>
<MDBBadge color="orange">
<MDBIcon fab icon="btc" size="2x" />
</MDBBadge>
<MDBBadge pill color="teal">
<MDBIcon icon="heart" size="2x" />
</MDBBadge>
<MDBBadge pill color="green">
<MDBIcon fab icon="apple" size="2x" />
</MDBBadge>
<MDBBadge pill color="purple">
<MDBIcon icon="users" size="2x" />
</MDBBadge>
</MDBContainer>
);
};
export default BadgePage;
Chips
Chips with avatars MDB Pro component
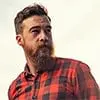
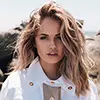
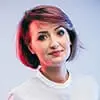
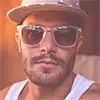
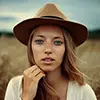
.webp)
import React from "react";
import { MDBChip, MDBContainer } from "mdbreact";
const BadgePage = () => {
return (
<MDBContainer>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-6.webp" alt="Contact Person" waves>
John Doe
</MDBChip>
<MDBChip size="md" src="https://mdbootstrap.com/img/Photos/Avatars/avatar-10.webp" alt="Contact Person" waves>
Anna Smith
</MDBChip>
<MDBChip size="lg" src="https://mdbootstrap.com/img/Photos/Avatars/avatar-5.webp" alt="Contact Person" waves>
Lara Lim
</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-8.webp" alt="Contact Person" bgColor="light-blue lighten-4"
waves>
Tom Dark
</MDBChip>
<MDBChip size="md" src="https://mdbootstrap.com/img/Photos/Avatars/avatar-12.webp" alt="Contact Person" bgColor="light-blue lighten-4"
waves>
Kate Horwitz
</MDBChip>
<MDBChip size="lg" src="https://mdbootstrap.com/img/Photos/Avatars/img(27).webp" alt="Contact Person" bgColor="light-blue lighten-4"
waves>
Danny Clark
</MDBChip>
</MDBContainer>
);
};
export default BadgePage;
Chips without avatars MDB Pro component
import React from "react";
import { MDBChip } from "mdbreact";
class BadgePage extends React.Component {
state = {
show1: true,
show2: true,
show3: true,
show4: true,
show5: true,
show6: true,
show7: true,
show8: true,
show9: true,
show10: true,
show11: true,
show12: true
}
handleCloseHere = (param) => (e) => {
this.setState({
['show' + param]: false
})
}
render() {
return (
<div>
{this.state.show1 && <MDBChip waves close handleClose={this.handleCloseHere(1)}> Tag 1 </MDBChip>}
{this.state.show2 && <MDBChip waves close handleClose={this.handleCloseHere(2)}> Tag 2 </MDBChip>}
{this.state.show3 && <MDBChip waves close handleClose={this.handleCloseHere(3)}> Tag 3 </MDBChip>}
{this.state.show4 && <MDBChip waves close handleClose={this.handleCloseHere(4)}> Tag 4 </MDBChip>}
{this.state.show5 && <MDBChip waves close handleClose={this.handleCloseHere(5)}> Tag 5 </MDBChip>}
{this.state.show6 && <MDBChip waves close handleClose={this.handleCloseHere(6)}> Tag 6 </MDBChip>}
{this.state.show7 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(7)}> Tag 220</MDBChip>}
{this.state.show8 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(8)}> Tag 219</MDBChip>}
{this.state.show9 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(9)}> Tag 218</MDBChip>}
{this.state.show10 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(10)}> Tag 217</MDBChip>}
{this.state.show11 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(11)}> Tag 216</MDBChip>}
{this.state.show12 && <MDBChip bgColor="pink lighten-4" waves close handleClose={this.handleCloseHere(12)}> Tag 215</MDBChip>}
</div>
)
}
}
export default BadgePage;
Colorful chips MDB Pro component
MDB has hundreds of colors to use within chips. Take a look here to know all the possibilities.
.webp)
.webp)
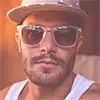
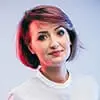
.webp)
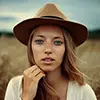
.webp)
.webp)
.webp)
import React from "react";
import { MDBChip, MDBCard } from "mdbreact";
class ChipsPage extends React.Component {
state = {
show13: true,
show14: true,
show15: true,
show16: true,
show17: true,
show18: true,
show19: true,
show20: true,
show21: true,
show22: true,
show23: true,
show24: true,
show25: true
}
handleCloseHere = param => e => {
this.setState({
["show" + param]: false
});
};
render() {
const { show13, show14, show15, show16, show17, show18, show19, show20, show21, show22, show23, show24, show25 } =
this.state;
return (
<MDBCard style={{ maxWidth: "65%", padding: "1.25rem" }}>
<div className="mb-4">
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/img(7).webp" alt="Contact Person" bgColor="blue lighten-4"
waves>
Caroline Smith
</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/img(3).webp" alt="Contact Person" bgColor="purple lighten-4"
waves>
Adam Grey
</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-8.webp" alt="Contact Person" bgColor="amber lighten-3"
waves>
Danny Moor
</MDBChip>
</div>
<div className="mb-4">
<MDBChip size="md" src="https://mdbootstrap.com/img/Photos/Avatars/avatar-5.webp" alt="Contact Person" bgColor="orange darken-2"
text="white" waves>
Daisy Sun
</MDBChip>
<MDBChip size="md" src="https://mdbootstrap.com/img/Photos/Avatars/img(28).webp" alt="Contact Person" bgColor="cyan darken-2"
text="white" waves>
Martha Lores
</MDBChip>
<MDBChip size="md" src="https://mdbootstrap.com/img/Photos/Avatars/avatar-12.webp" alt="Contact Person" bgColor="pink darken-2"
text="white" waves>
Alexandra Deyn
</MDBChip>
</div>
<div className="mb-4">
<MDBChip size="lg" src="https://mdbootstrap.com/img/Photos/Avatars/img(27).webp" alt="Contact Person" bgColor="primary-color"
text="white" waves>
Olaf Horwitz
</MDBChip>
<MDBChip size="lg" src="https://mdbootstrap.com/img/Photos/Avatars/img(30).webp" alt="Contact Person" bgColor="danger-color"
text="white" waves>
Mary-Kate Dare
</MDBChip>
<MDBChip size="lg" src="https://mdbootstrap.com/img/Photos/Avatars/img(21).webp" alt="Contact Person" bgColor="success-color"
text="white" waves>
The Sylvester
</MDBChip>
</div>
<div className="mb-4">
{show13 && (
<MDBChip bgColor="teal lighten-2" text="white" close waves handleClose={this.handleCloseHere(13)}>
Martha
</MDBChip>
)}
{show14 && (
<MDBChip bgColor="pink lighten-2" text="white" close waves handleClose={this.handleCloseHere(14)}>
Agnes
</MDBChip>
)}
{show15 && (
<MDBChip bgColor="light-blue lighten-2" text="white" close waves handleClose={this.handleCloseHere(15)}>
Caroline
</MDBChip>
)}
{show16 && (
<MDBChip bgColor="purple lighten-2" text="white" close waves handleClose={this.handleCloseHere(16)}>
Elisa
</MDBChip>
)}
{show17 && (
<MDBChip bgColor="mdb-color lighten-2" text="white" close waves handleClose={this.handleCloseHere(17)}>
Francesca
</MDBChip>
)}
</div>
<div className="mb-4">
{show18 && (
<MDBChip size="md" bgColor="red lighten-4" text="red" close waves handleClose={this.handleCloseHere(18)}>
25.09.2017
</MDBChip>
)}
{show19 && (
<MDBChip size="md" bgColor="indigo lighten-4" text="indigo" close waves handleClose={this.handleCloseHere(19)}>
24.08.2016
</MDBChip>
)}
{show20 && (
<MDBChip size="md" bgColor="cyan lighten-4" text="cyan" close waves handleClose={this.handleCloseHere(20)}>
23.07.2015
</MDBChip>
)}
{show21 && (
<MDBChip size="md" bgColor="deep-purple lighten-4" text="deep-purple" close waves handleClose={this.handleCloseHere(21)}>
22.06.2014
</MDBChip>
)}
</div>
<div className="mb-4">
{show22 && (
<MDBChip size="lg" gradient="aqua" text="white" close waves handleClose={this.handleCloseHere(22)}>
Aqua gradient
</MDBChip>
)}
{show23 && (
<MDBChip size="lg" gradient="peach" text="white" close waves handleClose={this.handleCloseHere(23)}>
Peach gradient
</MDBChip>
)}
{show24 && (
<MDBChip size="lg" gradient="purple" text="white" close waves handleClose={this.handleCloseHere(24)}>
Purple gradient
</MDBChip>
)}
{show25 && (
<MDBChip size="lg" gradient="blue" text="white" close waves handleClose={this.handleCloseHere(25)}>
Blue gradient
</MDBChip>
)}
</div>
</MDBCard>
);
};
}
export default ChipsPage;
Chip input MDB Pro component
Adding tags
Chip input is a component that helps to style and render collections of strings. It accepts
placeholder
String prop, that gets replaced with secondaryPlaceholder
, if
present after there is a chip in already. Try adding tags by hitting Enter and deleting them by
pressing
X.
import React from 'react';
import { MDBChipsInput } from 'mdbreact';
const ChipsInputPage = () => {
return (
<MDBChipsInput placeholder='+Tag' secondaryPlaceholder='Enter a tag' />
);
};
export default ChipsInputPage;
Setting initial tags
Chip input can use chips
Array prop to render predefined tags.
import React from 'react';
import { MDBChipsInput } from 'mdbreact';
const ChipsInputPage = () => {
return <MDBChipsInput chips={['Tag 1', 'Tag 2', 'Tag 3']} />;
};
export default ChipsInputPage;
React Tags - API
In this section you will find advanced information about the Tags component. You will learn which modules are required in this component, what are the possibilities of configuring the component, and what events and methods you can use in working with it.
Import statement
import { MDBBadge, MDBChip, MDBChipsInput } from "mdbreact";
API Reference: MDBBadge Properties
The table below shows the configuration options of the MDBBadge component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom class to the MDBBadge component | <MDBBadge className="myClass" /> |
tag |
String | 'span' |
Changes badge's wrapper tag | <MDBBadge tag="p" /> |
color |
String |
|
Changes badge's color | <MDBBadge color="primary" /> |
pill |
Boolean | false |
Changes badge into pill | <MDBBadge pill /> |
API Reference: MDBChip Properties
The table below shows the configuration options of the MDBChip component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom class to the MDBChip component | <MDBChip className="myClass" /> |
tag |
String |
|
Changes size of MDBChip component. Choose from lg | md | sm |
<MDBChip tag="p" /> |
size |
String | 'div' |
Changes MDBChip's wrapper tag. | <MDBChip size="lg" /> |
bgColor |
String |
|
Changes MDBChip's color | <MDBChip color="primary" /> |
text |
String |
|
Sets MDBChip's text color | <MDBChip text="white" /> |
gradient |
String |
|
Sets MDBChip's gradient style | <MDBChip gradient="aqua" /> |
src |
String |
|
Lets you to add img and sets it's src attribute | <MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-6.webp" /> |
alt |
String |
|
Defines img alt attribute | <MDBChip alt="my avatar" /> |
close |
Boolean |
|
Add close icon to your MDBChip and attaches to it onClick event which which fires custom handleCloseClick function. | <MDBChip close /> |
waves |
Boolean |
|
Adds waves effect to the MDBChip component. | <MDBChip waves /> |
handleClose |
function |
|
Lets you define custom function fired when close icon is clicked. | <MDBChip handleClose={this.myCloseFunction} /> |
API Reference: MDBChipsInput Properties
The table below shows the configuration options of the MDBChipsInput component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom class to the wrapper component | <MDBChipsInput className="myClass" /> |
tag |
String |
|
Changes MDBChipsInput's wrapper tag. | <MDBChipsInput tag="p" /> |
handleAdd |
function |
|
Returns an object with ID (index), value (content) and target (html element) of added MDBChipsInput's. | <MDBChipsInput handleAdd={(value) => console.log(value)} /> |
handleClose |
function |
|
Lets you define custom function fired when MDBChip close icon is clicked. | <MDBChipsInput handleClose={this.myCloseFunction} /> |
handleRemove |
function |
|
Returns an object with ID (index) and value (content) of removed MDBChipsInput's. | <MDBChipsInput handleRemove={(value) => console.log(value)} /> |
placeholder |
String |
|
Defines input placeholder | <MDBChipsInput placeholder="Custom placeholder" /> |
secondaryPlaceholder |
String |
|
Defines input secondary placeholder | <MDBChipsInput secondaryPlaceholder="Custom placeholder" /> |
chipSize |
String |
|
Changes MDBChip's size. Choose from lg |md |sm |
<MDBChipsInput chipSize="lg" /> |
chipColor |
String |
|
Changes MDBChip's color | <MDBChipsInput chipColor="cyan" /> |
chipText |
String |
|
Sets MDBChip's text color | <MDBChipsInput chipText="white" /> |
chipGradient |
String |
|
Sets MDBChip's gradient style | <MDBChipsInput chipGradient="aqua" /> |
chipGradient |
String |
|
Sets MDBChip's gradient style | <MDBChipsInput chipGradient="aqua" /> |
waves |
String |
|
Adds waves effect to the MDBChip component. | <MDBChipsInput waves /> |
chips |
Array |
|
Predefines tags to render | <MDBChipsInput chips={["Tag 1", "Tag 2", "Tag 3"]} /> |
getValue |
String |
|
Returns an array with actual list of MDBChipsInput's | <MDBChipsInput getValue={(value) => console.log(value)} /> |